Hi, How can I install Office on Mac 10.9.5? There has been a similar post before, but the answer leads to many upload limks and I can't find which one is the one to follow. Microsoft States Office for Mac 2016 requires OS X 10.10 or higher. Office 2016 is available either as a stand alone product or as part of an office 365 subscription from Microsoft. There is not and never was an 'Office for Mac 2013'. Jan 5, 2016 7:23 AM.
- Microsoft Office 2010 94fbr
- Microsoft Office For Mac Os 10.9.5
- Microsoft Office For Mac 10.9 5
- Microsoft Office 1099 Misc Template
C# has features that simplify access to Office API objects. The new features include named and optional arguments, a new type called dynamic
, and the ability to pass arguments to reference parameters in COM methods as if they were value parameters.
In this topic you will use the new features to write code that creates and displays a Microsoft Office Excel worksheet. You will then write code to add an Office Word document that contains an icon that is linked to the Excel worksheet.
To complete this walkthrough, you must have Microsoft Office Excel 2007 and Microsoft Office Word 2007, or later versions, installed on your computer.
Note
Your computer might show different names or locations for some of the Visual Studio user interface elements in the following instructions. The Visual Studio edition that you have and the settings that you use determine these elements. For more information, see Personalizing the IDE.
To create a new console application
Start Visual Studio.
On the File menu, point to New, and then click Project. The New Project dialog box appears.
In the Installed Templates pane, expand Visual C#, and then click Windows.
Look at the top of the New Project dialog box to make sure that .NET Framework 4 (or later version) is selected as a target framework.
In the Templates pane, click Console Application.
Type a name for your project in the Name field.
Click OK.
The new project appears in Solution Explorer.
To add references
Microsoft Office 2010 94fbr
In Solution Explorer, right-click your project's name and then click Add Reference. The Add Reference dialog box appears.
On the Assemblies page, select Microsoft.Office.Interop.Word in the Component Name list, and then hold down the CTRL key and select Microsoft.Office.Interop.Excel. If you do not see the assemblies, you may need to ensure they are installed and displayed. See How to: Install Office Primary Interop Assemblies.
Click OK.
To add necessary using directives
In Solution Explorer, right-click the Program.cs file and then click View Code.
Add the following
using
directives to the top of the code file:
To create a list of bank accounts
Paste the following class definition into Program.cs, under the
Program
class.Add the following code to the
Main
method to create abankAccounts
list that contains two accounts.
To declare a method that exports account information to Excel
Add the following method to the
Program
class to set up an Excel worksheet.Method Add has an optional parameter for specifying a particular template. Optional parameters, new in C# 4, enable you to omit the argument for that parameter if you want to use the parameter's default value. Because no argument is sent in the following code,
Add
uses the default template and creates a new workbook. The equivalent statement in earlier versions of C# requires a placeholder argument:ExcelApp.Workbooks.Add(Type.Missing)
.Add the following code at the end of
DisplayInExcel
. The code inserts values into the first two columns of the first row of the worksheet.Add the following code at the end of
DisplayInExcel
. Theforeach
loop puts the information from the list of accounts into the first two columns of successive rows of the worksheet.Add the following code at the end of
DisplayInExcel
to adjust the column widths to fit the content.Earlier versions of C# require explicit casting for these operations because
ExcelApp.Columns[1]
returns anObject
, andAutoFit
is an Excel Range method. The following lines show the casting.C# 4, and later versions, converts the returned
Object
todynamic
automatically if the assembly is referenced by the EmbedInteropTypes compiler option or, equivalently, if the Excel Embed Interop Types property is set to true. True is the default value for this property.
To run the project
Add the following line at the end of
Main
.Press CTRL+F5.
An Excel worksheet appears that contains the data from the two accounts.
To add a Word document
To illustrate additional ways in which C# 4, and later versions, enhances Office programming, the following code opens a Word application and creates an icon that links to the Excel worksheet.
Paste method
CreateIconInWordDoc
, provided later in this step, into theProgram
class.CreateIconInWordDoc
uses named and optional arguments to reduce the complexity of the method calls to Add and PasteSpecial. These calls incorporate two other new features introduced in C# 4 that simplify calls to COM methods that have reference parameters. First, you can send arguments to the reference parameters as if they were value parameters. That is, you can send values directly, without creating a variable for each reference parameter. The compiler generates temporary variables to hold the argument values, and discards the variables when you return from the call. Second, you can omit theref
keyword in the argument list.The
Add
method has four reference parameters, all of which are optional. In C# 4.0 and later versions, you can omit arguments for any or all of the parameters if you want to use their default values. In C# 3.0 and earlier versions, an argument must be provided for each parameter, and the argument must be a variable because the parameters are reference parameters.The
PasteSpecial
method inserts the contents of the Clipboard. The method has seven reference parameters, all of which are optional. The following code specifies arguments for two of them:Link
, to create a link to the source of the Clipboard contents, andDisplayAsIcon
, to display the link as an icon. In C# 4.0 and later versions, you can use named arguments for those two and omit the others. Although these are reference parameters, you do not have to use theref
keyword, or to create variables to send in as arguments. You can send the values directly. In C# 3.0 and earlier versions, you must supply a variable argument for each reference parameter.In C# 3.0 and earlier versions of the language, the following more complex code is required.
Add the following statement at the end of
Main
.Add the following statement at the end of
DisplayInExcel
. TheCopy
method adds the worksheet to the Clipboard.Press CTRL+F5.
A Word document appears that contains an icon. Double-click the icon to bring the worksheet to the foreground.
To set the Embed Interop Types property
Additional enhancements are possible when you call a COM type that does not require a primary interop assembly (PIA) at run time. Removing the dependency on PIAs results in version independence and easier deployment. For more information about the advantages of programming without PIAs, see Walkthrough: Embedding Types from Managed Assemblies.
In addition, programming is easier because the types that are required and returned by COM methods can be represented by using the type
dynamic
instead ofObject
. Variables that have typedynamic
are not evaluated until run time, which eliminates the need for explicit casting. For more information, see Using Type dynamic.In C# 4, embedding type information instead of using PIAs is default behavior. Because of that default, several of the previous examples are simplified because explicit casting is not required. For example, the declaration of
worksheet
inDisplayInExcel
is written asExcel._Worksheet workSheet = excelApp.ActiveSheet
rather thanExcel._Worksheet workSheet = (Excel.Worksheet)excelApp.ActiveSheet
. The calls toAutoFit
in the same method also would require explicit casting without the default, becauseExcelApp.Columns[1]
returns anObject
, andAutoFit
is an Excel method. The following code shows the casting.To change the default and use PIAs instead of embedding type information, expand the References node in Solution Explorer and then select Microsoft.Office.Interop.Excel or Microsoft.Office.Interop.Word.
If you cannot see the Properties window, press F4.
Find Embed Interop Types in the list of properties, and change its value to False. Equivalently, you can compile by using the References compiler option instead of EmbedInteropTypes at a command prompt.
To add additional formatting to the table
Replace the two calls to
AutoFit
inDisplayInExcel
with the following statement.The AutoFormat method has seven value parameters, all of which are optional. Named and optional arguments enable you to provide arguments for none, some, or all of them. In the previous statement, an argument is supplied for only one of the parameters,
Format
. BecauseFormat
is the first parameter in the parameter list, you do not have to provide the parameter name. However, the statement might be easier to understand if the parameter name is included, as is shown in the following code.Press CTRL+F5 to see the result. Other formats are listed in the XlRangeAutoFormat enumeration.
Compare the statement in step 1 with the following code, which shows the arguments that are required in C# 3.0 and earlier versions.
Example
The following code shows the complete example.
See also
The last OpenOffice version supporting Mac OS X 10.4 (Tiger), 10.5 (Leopard), 10.6 (Snow Leopard) is OpenOffice 4.0.1. Hardware Requirements ¶ CPU: Intel Processor. Aug 17, 2011 Old Version of LibreOffice for Mac for Mac OS X 10.5 Leopard (PowerPC) tead. LibreOffice is a free software office suite developed by The Document Foundation as a fork of OpenOffice.org. It is compatible with other major office suites, including Microsoft Office, and available on a variety of platforms.
Microsoft Office 2008 for Mac is a version of the Microsoft Office productivity suite for Mac OS X. It supersedes Office 2004 for Mac (which did not have Intel native code) and is the Mac OS X equivalent of Office 2007. Office 2008 was developed by Microsoft's Macintosh Business Unit and released on. The following instructions step through the process of configuring your Office 365 Exchange Online account with Apple Mail for Mac OS X 10.9 - 10.10. Step Open Apple Mail.
Microsoft Office 2008 for Mac applications: Word, Excel, PowerPoint and Entourage on Mac OS X 10.5 Leopard | |
Developer(s) | Microsoft |
---|---|
Initial release | January 15, 2008; 12 years ago |
Stable release | |
Operating system | Mac OS X 10.4.9 or later |
Type | Office suite |
License | |
Website | www.microsoft.com/mac/products/Office2008/default.mspx |
System requirements[2] | |
---|---|
CPU | PowerPC G4 or G5 (500 MHz or faster) or any Intel processor |
Operating system | Mac OS X10.4.9 or later |
RAM | 512 MB |
Free hard disk space | 1.5 GB |
Optical drive | DVD-ROM (for local installation) |
Notes | Unofficially runs on PowerPC G3 Macs (like the iMac G3 in Bondi Blue) and with less RAM |
Sep 07, 2017 Double-click the file that you downloaded in step 5 to place the Microsoft Office 2011 14.7.7 Update volume on your desktop, and then double-click the Microsoft Office 2011 14.7.7 Update volume to open it. This step might have been performed for you. Jul 28, 2019 Microsoft Office. I just bought Microsoft Office 2019 for my OS X 10.9.5 Mac. I downloaded the setup instructions but when I try to open it, it says Microsoft Windows applications are not supported on read more. Jun 12, 2019 On the Microsoft Store - Office FAQ page, there is a 'Can I install Office on my Mac?' Question under 'Office Download and Install'. It says, in part. If you have an active Office 365 Home, Personal or University subscription, and Mac OS X 10.10, you can install Office 2016 for Mac. If you have an older version of Mac OS X, you will.
Microsoft Office 2008 for Mac is a version of the Microsoft Officeproductivity suite for Mac OS X. It supersedes Office 2004 for Mac (which did not have Intel native code) and is the Mac OS X equivalent of Office 2007. Office 2008 was developed by Microsoft's Macintosh Business Unit and released on January 15, 2008. Office 2008 was followed by Microsoft Office for Mac 2011 released on October 26, 2010, requiring a Mac with an Intel processor and Mac OS version 10.5 or better. Office 2008 is also the last version to feature Entourage, which was replaced by Outlook in Office 2011. Microsoft stopped supporting Office 2008 on April 9, 2013.
Release[edit]
Office 2008 was originally slated for release in the second half of 2007; however, it was delayed until January 2008, purportedly to allow time to fix lingering bugs.[3] Office 2008 is the only version of Office for Mac supplied as a Universal Binary.
Unlike Office 2007 for Windows, Office 2008 was not offered as a public beta before its scheduled release date.[4]

Features[edit]
Office 2008 for Mac includes the same core programs currently included with Office 2004 for Mac: Entourage, Excel, PowerPoint and Word.
Mac-only features included are a publishing layout view, which offers functionality similar to Microsoft Publisher for Windows, a 'Ledger Sheet mode' in Excel to ease financial tasks, and a 'My Day' application offering a quick way to view the day's events.[5]
Office 2008 supports the new Office Open XML format, and defaults to saving all files in this format. On February 21, 2008 Geoff Price revealed that the format conversion update for Office 2004 would be delayed until June 2008 in order to provide the first update to Office 2008.[6]
Microsoft Visual Basic for Applications is not supported in this version.[7] As a result, such Excel add-ins dependent on VBA, such as Solver, have not been bundled in the current release.[8] In June 2008, Microsoft announced that it is exploring the idea of bringing some of the functionality of Solver back to Excel.[9] In late August 2008, Microsoft announced that a new Solver for Excel 2008 was available as a free download from Frontline Systems, original developers of the Excel Solver.[10][11] However, Excel 2008 also lacks other functionality, such as Pivot Chart functionality, which has long been a feature in the Windows version. In May 2008, Microsoft announced that VBA will be making a return in the next version of Microsoft Office for Mac.[12]AppleScript and the Open Scripting Architecture will still be supported.
Limitations[edit]
Office 2008 for Mac lacks feature parity with the Windows version. The lack of Visual Basic for Applications (VBA) support in Excel makes it impossible to use macros programmed in VBA. Microsoft's response is that adding VBA support in Xcode would have resulted in an additional two years added to the development cycle of Office 2008.[13] Other unsupported features include: OMML equations generated in Word 2007 for Windows,[14] Office 'Ribbon', Mini Toolbar, Live Preview, and an extensive list of features are unsupported such as equivalent SharePoint integration with the Windows version. Some features are missing on Excel 2008 for Mac, including: data filters (Data Bars, Top 10, Color-based, Icon-based), structured references, Excel tables, Table styles, a sort feature allowing more than three columns at once and more than one filter on a sort.
Benchmarks suggest that the original release of Office 2008 runs slower on Macs with PowerPC processors, and does not provide a significant speed bump for Macs with Intel processors.[15]
A using a program to remove application support files in unwanted languages), and which do not affect Office's operations, but which cause the updaters' installers to believe that the application is not valid for update. A small modification to the installer has been found an effective work-around (see reference).[18]
Another widespread problem reported after SP1 is that Office files will no longer open in Office applications when opened (double-clicked) from the Mac OS X Finder or launched from other applications such as an email attachment. The trigger for this problem is that Microsoft in SP1 unilaterally and without warning deprecated certain older Mac OS 'Type' codes such as 'WDBN' that some files may have, either because they are simply very old, or because some applications assign the older Type code when saving them to the disk. Users have seen the problem affect even relatively new Type codes, however, such as 'W6BN'. Microsoft is apparently looking into the problem, but it is unclear if they will reinstate the older Type codes, citing security concerns.[19]
Another problem with cross-platform compatibility is that images inserted into any Office application by using either cut and paste or drag and drop result in a file that does not display the inserted graphic when viewed on a Windows machine. Instead, the Windows user is told 'QuickTime and a TIFF (LZW) decompressor are needed to see this picture'. A user presented one solution as far back as December 2004.[20]
A further example of the lack of feature parity is the track changes function. Whereas users of Word 2003 or 2007 for Windows are able to choose freely between showing their changes in-line or as balloons in the right-hand margin,[21][22] choosing the former option in Word 2004 or Word 2008 for Mac OS also turns off all comment balloons; comments in this case are visible only in the Reviewing Pane or as popup boxes (i.e. upon mouseover).[23] This issue has not been resolved to date and is present in the latest version of Word for the Mac, namely Word 2011.[24]
The toolbox found in Office 2008 also has problems when the OS X feature Spaces is used: switching from one Space to another will cause elements of the Toolbox to get trapped on one Space until the Toolbox is closed and reopened. The only remedy for this problem is to currently disable Spaces, or at least refrain from using it whilst working in Office 2008.[25] Microsoft has acknowledged this problem and states that it is an architectural problem with the implementation of Spaces. Apple has been informed of the problem, according to Microsoft.[26] The problem appears to be caused by the fact that the Toolbox is Carbon-based.[citation needed] Using Microsoft Office with Mac OS X 10.6 Snow Leopard solves some of the problems.[26]
In addition, there is no support for right to left and bidirectional languages (such as Arabic, Hebrew, Persian, etc.) in Office 2008,[27][28] making it impossible to read or edit a right to left document in Word 2008 or PowerPoint 2008. Languages such as Thai are similarly not supported, although installing fonts can sometimes allow documents written in these languages to be displayed.
Moreover, Office 2008 proofing tools support only a limited number of languages (Danish, Dutch, English, Finnish, French, German, Italian, Japanese, Norwegian, Portuguese, Spanish, Swedish, and Swiss German).[29] Proofing tools for other languages failed to find their way to the installation pack, and are not offered by Microsoft commercially in the form of separately sold language packs. At the same time, Office applications are not integrated with the proofing tools native to Mac OS X 10.6 Leopard.
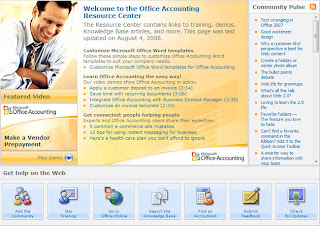
Microsoft Visio is not available for OS X. This means that any embedded Visio diagrams in other Office documents (e.g. Word) cannot be edited in Office on the Mac. Embedded Visio diagrams appear as a low-quality bitmap both in the WYSIWYG editor and upon printing the document on the Mac.
Editions[edit]
Applications and services | Home & Student | Standard | Business Edition | Special Media Edition |
---|---|---|---|---|
Word | Yes | Yes | Yes | Yes |
PowerPoint | Yes | Yes | Yes | Yes |
Excel | Yes | Yes | Yes | Yes |
Entourage | Yes | Yes | Yes | Yes |
Exchange Server support | No | Yes | Yes | Yes |
Automator Actions | No | Yes | Yes | Yes |
Office Live and SharePoint support | No | No | Yes | No |
Expression Media | No | No | No | Yes |
See also[edit]
References[edit]
- ^'Microsoft Support Lifecycle - Office 2008'. Microsoft. Retrieved February 10, 2018.
- ^'Microsoft Office 2008 for Mac Specs'. CNET. January 15, 2008. Retrieved January 5, 2017.
- ^'It's Coming: Mac BU Announces Intent to Deliver Office 2008 for Mac'. Microsoft. January 9, 2007. Archived from the original on October 11, 2007.
- ^'Microsoft Office 2008 for the Mac delayed until January 2008'. TUAW. August 2, 2007.
- ^'Microsoft starts testing Office 2008 for Mac'. Cnet. April 2, 2007. Archived from the original on September 28, 2007. Retrieved September 19, 2007.
- ^'MS Office Mac Discussion Board'. January 15, 2008.
- ^'Saying goodbye to Visual Basic'. August 8, 2006.
- ^'MS Office Mac Discussion Board'. January 15, 2008.
- ^'Excel 2008 and Solver'. June 26, 2008.
- ^'Solver For Excel 2008 Is Available'. August 29, 2008.
- ^'Solver is Back for Microsoft Excel 2008 on Macintosh'. August 29, 2008.
- ^'Microsoft Office Update, and Visual Basic for Applications to Return - Mac Rumors'. May 13, 2008.
- ^'MS Mactopia Blog'. March 13, 2008.
- ^Known issues in Word 2008 – Equations saved from Word 2007 for Windows do not appear in Word 2008 for Mac
- ^'MS Mactopia Blog'. March 13, 2008.
- ^'CambridgeSoft Website'.
- ^New installer for 12.0.1 (The Entourage Help Blog)
- ^MacFixit article: More Fixes for Problems InstallingArchived January 26, 2009, at the Wayback Machine
- ^http://www.microsoft.com/mac/help.mspx?target=0b9aa757-50ab-443b-8b0e-3a50ece1d5451033&clr=99-4-0
- ^'Archived copy'. Archived from the original on June 26, 2008. Retrieved June 30, 2008.CS1 maint: archived copy as title (link)
- ^'Archived copy'. Archived from the original on July 2, 2009. Retrieved July 9, 2009.CS1 maint: archived copy as title (link)
- ^'IT training – IT training – IT Services – Administrative and academic support divisions – Services and divisions – Staff and students – Home'. Ittraining.lse.ac.uk. May 7, 2010. Archived from the original on February 27, 2009. Retrieved May 30, 2010.
- ^[1][dead link]
- ^http://officeformac.com/ms/ProductForums/Word/11634/0
- ^Bugs & Fixes: Office 2008 and Leopard’s Spaces don’t mix, Macworld, December 8, 2008
- ^ abOffice 2008 for Mac and Mac OS X Spaces, Microsoft
- ^Help and How-To for Microsoft for Mac Office Products Mactopia
- ^Higgaion » It’s official: no RTL support in Microsoft Office 2008 for Mac
- ^Proofing tools that are available for each language
External links[edit]
- MacBU interview: Office 2008 Exchange Server support[permanent dead link]
The Mac OS X Mavericks 10.9 release proved to be the most significant of all the releases made by apple in the Mac operating system X series. Mavericks proved to have a lot of in-built features that made it stand apart from all other operating systems released under the tag of the Mac OS X line up. The major standpoint behind the release of the Mavericks OS was t make sure that there was increased battery life, more adaptability and add in more application and revamp the old applications in terms of aesthetics.
License
Official Installer
File Size
5.0GB / 5.1GB
Language
English
Developer
Apple Inc.
Overview
The Mavericks OS also made sure that the mac system was stable in terms of performance and made the best out of the existing software such that apple didn’t have to run the compatibility test on the newly developed software. The OS also packed powerful improvements in terms of graphical efficiency as well as to get the most out of your RAM. All these elements packed together made sure that the Mac OS X Mavericks got a superior hold over the battery performance.
There are also various other features like the improvement in the usage of the applications through aesthetic detailing as well as user-friendliness embedded into the Mac OS makes it even more appealing.
1. iBooks
The iBooks is a beautifully done software that is exclusively built for reading books. This application covers all the aspects of a perfect reading application starting from night mode reading to exclusive fonts, highlighting and taking notes and much more. There is a wide range of collections in the library that allows you to have nearly 2 million book collections. Some of the basic things that come along with the system are the syncing of all the mac devices so that all the books that you register using the Apple device will be available throughout all the devices that are connected across along all the devices.
Microsoft Office For Mac Os 10.9.5
2. Multiple Display support
Office 365 mac. There was an expectation among the mac users that they wanted to have the extensions of their macs to other displays as well. This was particularly the request from all the graphical designers and people whose life revolved around the heavy usage of monitors and computers. Apple decided to release this feature along with the Mac OS X mavericks to ensure that the need was looked into. It did not require much of the configurations to enable this display configuration. The extensions of the display were just like that done as an extension to other system displays that necessarily didn’t have to be an apple monitor. This also made sure that the graphical card was put to the best use.
Microsoft Office For Mac Os X 10.9 5 Download
3. Apple maps
The much-anticipated application from apple was Apple Maps. It is a dedicated map application that was designed for Apple devices to get the best out of your outside world. Apple maps had all the features of a conventional map application with all the revolutionary standpoints that would be covered by a map application. The apple map also displayed adaptive such that the application would adjust the resolution accordingly to the device it is being used in.
Apple decided to release apple maps with the Mac OS X Mavericks keeping in mind that the OS was the revolutionary kind that was released with all the standard features that will remain in Apple devices.
4. Apple calendar
Microsoft Office For Mac 10.9 5
The apple calendar was also a feature that was adored by many with the release of the Mac OS X Mavericks. The apple calendar will help you with events and also it will help you integrate the newly added maps application to get your way to a mentioned event in the calendar.
5. iCloud keychain
The iCloud keychain was released along with the Mac OS X Mavericks as there was an update in the framework of Safari to support the keychain feature. The iCloud Keychain is a password keeper that is embedded along with the Mac OS to ensure that all your passwords are kept safe and secure. The Security protocol of iCloud Keychain is so complex that it would definitely be a tough framework to crack into. It saves information starting from credit card information to the Wi-Fi logins as well. This made sure that the Mac OS X Mavericks was much more user-friendly as well with its release.
System Requirements
- Minimum 2GB of RAM
- Minimum of 8GB HDD drive
- It can run on any mac system that can run the OS X mountain lion.
Download the MAC OS X Mavericks 10.9 ISO and DMG File
The mac OS X Mavericks are one of the most revolutionary Mac OS X releases that imbibed all the revolutionary features that make apple stand a class apart. you can download the DMG file from the below link and make sure that you have met all the basic system that requires to run the Mac OS X Mavericks on your Mac system without any hassles.
Mac OS X Mavericks 10.9 ISO and DMG Image Download
The Mac OS X Mavericks 10.9 release proved to be the most significant of all the releases made by apple in the Mac operating system X series. Mavericks proved to have a lot of in-built features that made it stand apart from all other operating systems released under the tag of the Mac OS X line up. The major standpoint behind the release of the Mavericks OS was t make sure that there was increased battery life, more adaptability and add in more application and revamp the old applications in terms of aesthetics.
Price Currency: USD
Operating System: Mac OS X Mavericks 10.9
Microsoft Office For Mac Os
Application Category: OS
Microsoft Office 1099 Misc Template
Microsoft Office For Mac Os X 10.9 5 Free
5